Carbon Fields VS ACF PRO Gutenberg blocks
This article compares Carbon Fields and ACF PRO for building Gutenberg blocks in WordPress. It highlights their features, performance, advantages, and drawbacks, helping developers choose the best tool for their custom block needs.
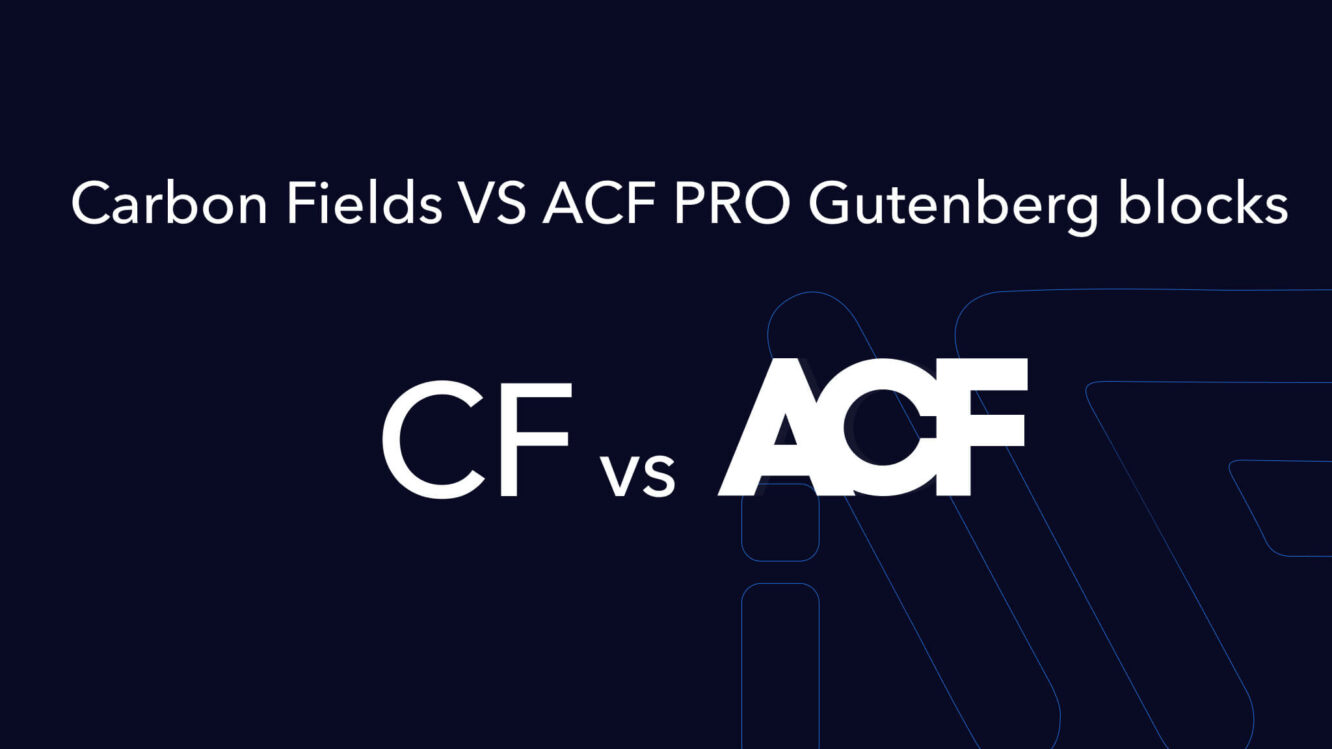
Carbon Fields is a WordPress library for creating and managing custom fields using a simple API. It allows developers to easily add fields to posts, taxonomies, users, comments, and widgets. It supports Gutenberg blocks and repeating fields.
Carbon Fields is free, open-source, compatible with PHP 5.3+, and suitable for commercial projects. It also supports metafields and dynamic interfaces for enhanced functionality.
If you develop themes using OOP and automatic class loading, you’ll find Carbon Fields easy to work with. It can be installed with Composer.
Pros of Carbon Fields:
- There is no admin panel interface for field creation, meaning fields are only created via code, reducing the chance for clients to rename fields and break the code accidentally.
- A large variety of pre-built field types.
- Flexibility to extend and create custom fields.
- Excellent documentation with examples.
- Free, open-source, and usable in any commercial project.
- It supports older PHP versions and is fully compatible with PHP 8.3.
Cons of Carbon Fields:
- Requires knowledge of OOP for field implementation.
- Installed via composer, which some developers may not be familiar with. (If you need help, read this article on how to use composer in your theme or plugin.)
- The incomplete interface for Gutenberg blocks only shows fields without a visual preview like ACF PRO.
Step-by-step Code for Connecting Carbon Fields
We will start by initializing the composer in the theme directory by running the composer init command and answering a few questions.
After that, the vendor
folder and the composer.json file should be created. Next, install Carbon Fields by running:
composer require htmlburger/carbon-fields
Add the following code in the functions.php file to load the main library file:
<?php
/**
* Connect library startup file
*
* @return void
*/
function crb_load() {
require_once('vendor/autoload.php');
\Carbon_Fields\Carbon_Fields::boot();
}
add_action('after_setup_theme', 'crb_load');
Code to Create a Gutenberg Block in Carbon Fields
<?php
/**
* Add Block FAQ.
*/
function crb_add_gutenberg_block() {
// FAQ block.
Block::make(
__( 'FAQ', 'iwpdev' )
)->add_fields(
[
Field::make(
'complex',
'faq_items',
__( 'FAQs', 'iwpdev' )
)->add_fields(
[
Field::make( 'text', 'title', __( 'Title', 'iwpdev' ) ),
Field::make( 'rich_text', 'description', __( 'Description', 'iwpdev' ) ),
]
),
]
)->set_category(
'iwpdev',
'editor-code'
)->set_render_callback(
function ( $fields, $attributes, $inner_blocks ) {
get_template_part(
'template-parts/blocks/faq',
'block',
[
'attributes' => $attributes,
'fields' => $fields,
]
);
}
);
}
add_action( 'carbon_fields_register_fields', 'crb_add_gutenberg_block' );
Code for Enqueuing Bootstrap 5 Styles and Scripts
<?php
/**
* Add Bootstrap 5
*
* @return void
*/
function add_bootstrap() {
wp_enqueue_style('iwp_bootstrap', '//cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css', [], '5.2.3');
wp_enqueue_script('iwp_bootstrap', '//cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js', ['jquery'], '5.2.3', true);
}
add_action('wp_enqueue_scripts', 'add_bootstrap');
Block Template Code
<?php
$fields = $args['fields'];
$attributes = $args['attributes'];
if ( ! empty( $fields['faq_items'] ) ) {
?>
<div class="accordion" id="accordionPanelsStayOpenExample">
<?php foreach ( $fields['faq_items'] as $key => $item ) { ?>
<div class="accordion-item">
<h2 class="accordion-header">
<button class="accordion-button collapsed" type="button" data-bs-toggle="collapse"
aria-expanded="false"
data-bs-target="#<?php echo esc_html( 'accord-' . $key ); ?>">
<?php echo esc_html( $item['title'] ?? '' ); ?>
</button>
</h2>
<div id="<?php echo esc_html( 'accord-' . $key ); ?>" class="accordion-collapse collapse"
aria-labelledby="panelsStayOpen-headingOne">
<div class="accordion-body">
<?php echo wp_kses_post( wpautop( $item['description'] ?? '' ) ); ?>
</div>
</div>
</div>
<?php } ?>
</div>
<?php
}
At this point, our Gutenberg block should appear as shown in the screenshots.
Results We Obtained:
- Page Generation Time: 0.2100s
- Peak Memory Usage: 6.7 MB
- Database Queries: 32
- Database Queries Time: 0.0104s
- Number of Scripts Loaded: 10
- Number of Styles Loaded: 24
/**
* FAQ register block.
*
* @return void
*/
function register_gutenberg_blocks() {
if (function_exists('acf_register_block')) {
acf_register_block_type([
'name' => 'FAQ',
'title' => __('FAQ', 'iwpdev'),
'description' => __('FAQ', 'iwpdev'),
'category' => 'acf-blocks',
'post_types' => ['page'],
'supports' => ['anchor' => true, 'align' => ['full', 'wide'], 'jsx' => true],
'render_template' => 'template-parts/block/faq.php',
]);
}
}
add_action('acf/init', 'register_gutenberg_blocks');
We create the block in the admin panel using one repeater field with two subfields: one for the title and the other for the content.
Don’t forget to select the template rendering option for this block, which was registered in the code above.
If everything is done correctly, you should see this block appear as shown in the screenshots.
Block Template Code
<?php
$faq_arg = get_field('faq');
if (!empty($faq_arg)) { ?>
<div class="accordion" id="accordionPanelsStayOpenExample">
<?php foreach ($faq_arg as $key => $item) { ?>
<div class="accordion-item">
<h2 class="accordion-header">
<button class="accordion-button collapsed" type="button" data-bs-toggle="collapse" aria-expanded="false" data-bs-target="#<?php echo esc_html('accord-' . $key); ?>">
<?php echo esc_html($item['title'] ?? ''); ?>
</button>
</h2>
<div id="<?php echo esc_html('accord-' . $key); ?>" class="accordion-collapse collapse" aria-labelledby="panelsStayOpen-headingOne">
<div class="accordion-body">
<?php echo wp_kses_post(wpautop($item['description'] ?? '')); ?>
</div>
</div>
</div>
<?php } ?>
</div>
<?php }
Results We Obtained with ACF PRO:
- Page Generation Time: 0.4336s
- Peak Memory Usage: 8.1 MB
- Database Queries: 38
- Database Queries Time: 0.0109s
- Number of Scripts Loaded: 8
- Number of Styles Loaded: 24
Parameter name | ACF | Carbone Fields |
Page Generation Time | – | + |
Peak Memory Usage | – | + |
Database Queries | – | + |
Database Queries Time | – | + |
Number of Scripts Loaded | + | – |
Number of Styles Loaded | + | + |
Speed of field creation | + | – |
Conclusion and Full Comparison
Carbon Fields is faster and more lightweight, especially if your theme contains many custom blocks, which provides a small boost in speed. It works reliably on both older and newer versions of PHP. Clients cannot change field parameters, ensuring the stability of your code, which is crucial for large projects.
ACF is an excellent solution for quickly creating blocks and implementing them without much coding knowledge. However, the downside is the cost and longer block generation times on the front end.
Ready to Take Your Project
to the Next Level?
Let’s bring your vision to life with expert development and custom solutions.
Contact us now and get started on transforming your ideas into reality!